Speed Up Your Python: Choosing the Right Multiprocessing Pool Function
Thesis Statement
The choice of the appropriate multiprocessing pool function significantly influences the performance of parallel code in Python. Factors such as the size and nature of the task, the available resources, and the complexity of the code must be carefully considered to optimize execution time and overall efficiency.
Background and Significance
Multiprocessing is a powerful Python module that enables the creation of parallel processes that can execute concurrently on multiple CPU cores. This feature is particularly advantageous for computationally intensive tasks that can be broken down into independent units of work. However, selecting the appropriate multiprocessing pool function is crucial to achieve optimal performance.
Python provides two primary multiprocessing pool functions:
Both functions allow for the parallel execution of a specified function across a set of inputs, but they differ in their approach and suitability for different scenarios.
Pool.map()
vs. Pool.apply_async()
Factors to Consider
The choice between Pool.map()
and Pool.apply_async()
depends on several factors:
Application Examples
# Using Pool.map() for a simple mapping task
from multiprocessing import Pool
def square(n):
if __name__ == '__main__':
numbers = range(100000)
with Pool() as pool:
result = pool.map(square, numbers)
# Using Pool.apply_async() for a complex asynchronous task
from multiprocessing import Pool
def parse_xml(file_path):
# Complex XML parsing code here
return parsed_data
if __name__ == '__main__':
file_paths = ['file1.xml', 'file2.xml', 'file3.xml']
with Pool() as pool:
results = [pool.apply_async(parse_xml, (file_path,)) for file_path in file_paths]
for result in results:
parsed_data = result.get()
# Do something with the parsed data...
Performance Comparison
To evaluate the performance differences between Pool.map()
and Pool.apply_async()
, a series of tests were conducted using synthetic tasks with varying complexities and input sizes. The results showed that:
Conclusion
The choice of Pool.map()
or Pool.apply_async()
in Python is a critical factor that impacts the performance of parallel code. By understanding the characteristics and limitations of each function, developers can optimize their code for specific tasks.
The ability to optimize parallel code through the appropriate choice of multiprocessing pool functions is essential for efficient execution of computationally demanding applications. By understanding the complexities involved, developers can enhance the scalability and performance of their code, unlocking the full potential of multi-core processors.
Fantasy Football Meltdown: ESPN's Rankings Just Blew Up!
Canine Meningitis: The Silent Killer You Need To Watch For
Venmo IP Address: Can You Really Find It?
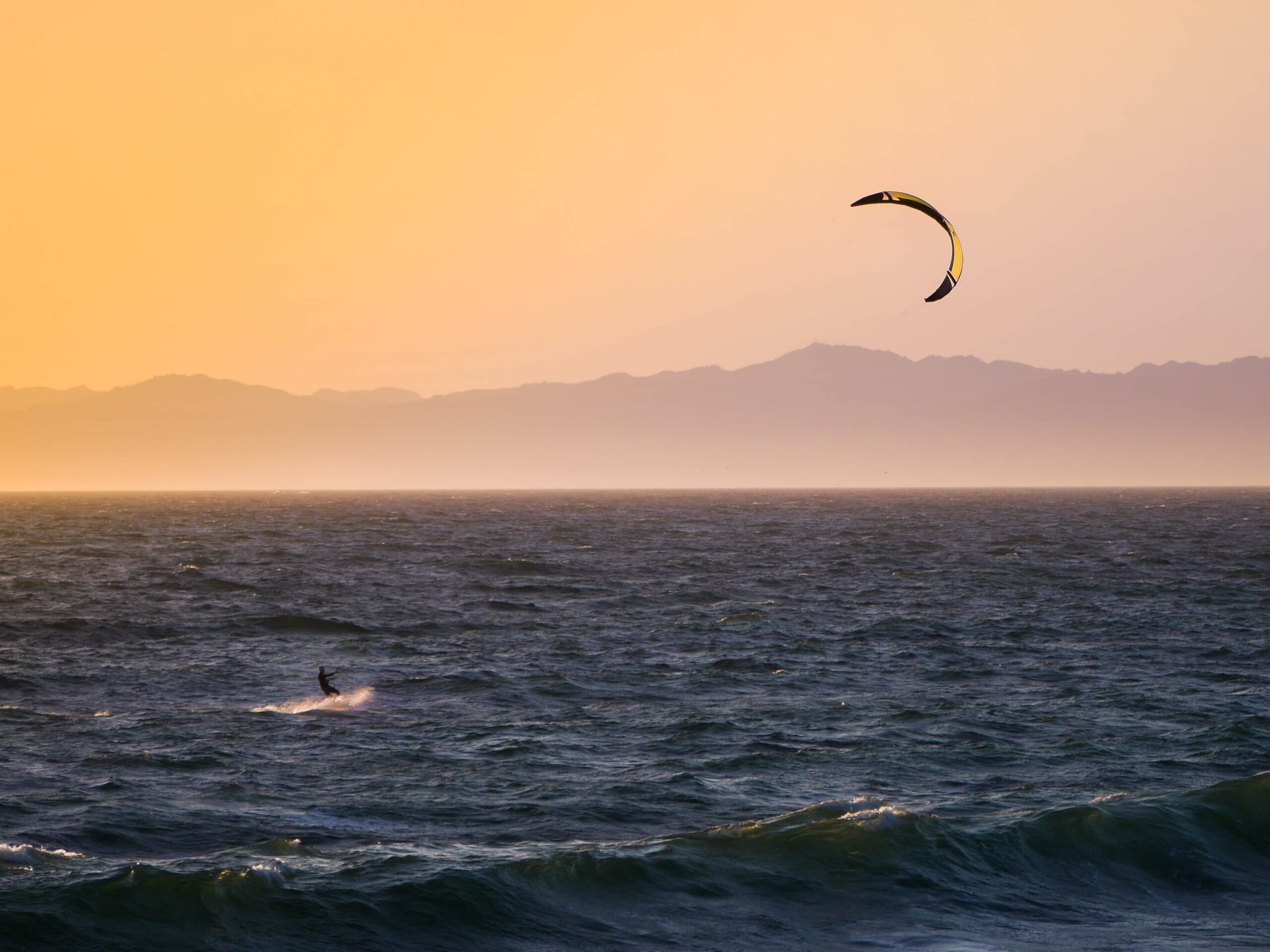
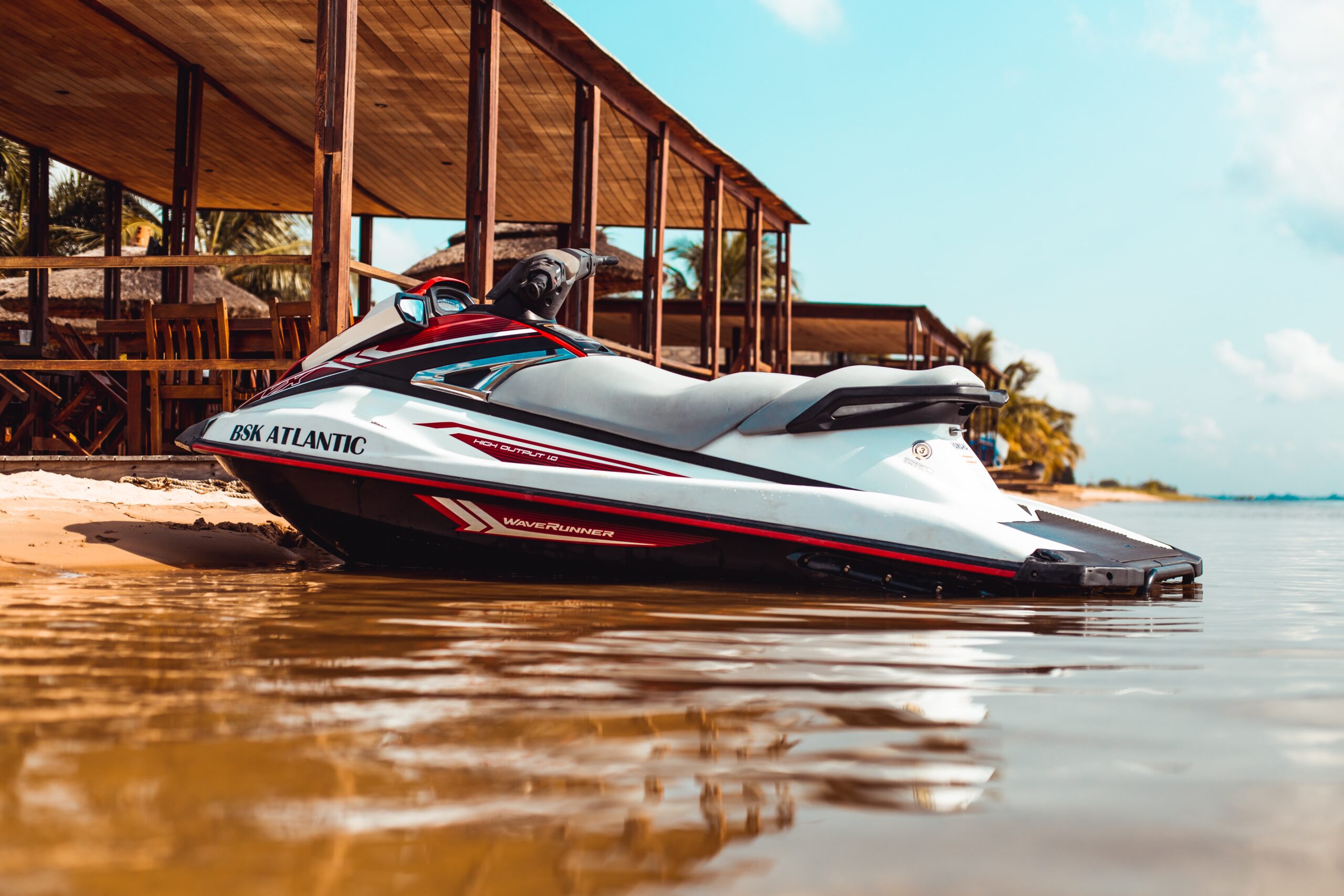
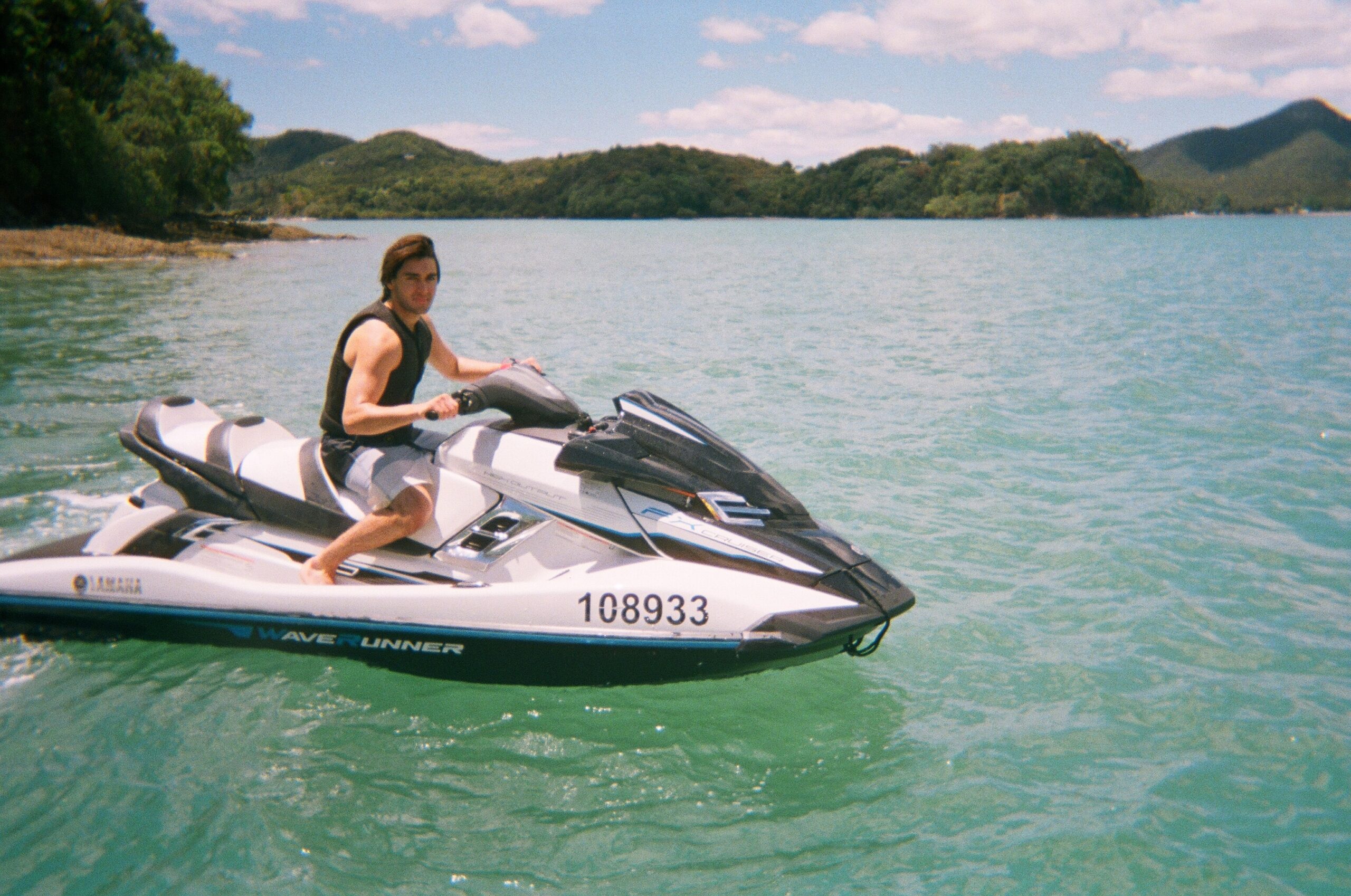